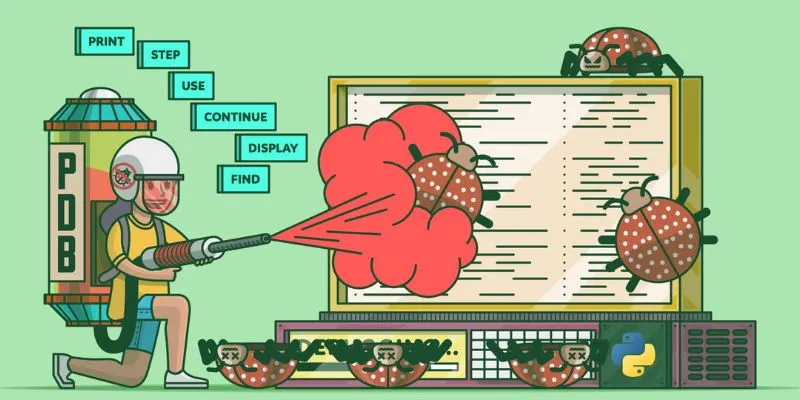
Debugging is an essential part of the software development process, and one of the simplest methods to debug Python code is by using print statements. Print debugging might seem basic, but it’s an effective way to track down issues in your code, especially in smaller projects or quick troubleshooting sessions. For those looking to deepen their debugging skills and overall Python proficiency, consider enrolling in a Python Course in Chennai, where you can learn best practices and advanced debugging techniques alongside the fundamentals.
1. Trace Variable Values
One of the key ways to use print statements is by printing out the values of variables at different points in your code. By inserting print statements, you can see whether variables are being assigned the correct values or if unexpected behavior is occurring.
x = 5
y = 10
print(f”Before operation: x = {x}, y = {y}”)
z = x + y
print(f”After operation: z = {z}”)
2. Track Program Flow
When dealing with complex programs or functions that have multiple execution paths, print statements can help you trace the flow of execution. Placing print statements in strategic locations allows you to verify if certain parts of your code are being executed as expected.
def check_value(val):
if val > 10:
print(“Value is greater than 10”)
elif val == 10:
print(“Value is exactly 10”)
else:
print(“Value is less than 10”)
check_value(15)
3. Identify Logic Errors
Sometimes, the code runs without errors but doesn’t produce the desired result due to a logic flaw. Print statements help identify where the logic breaks down by showing intermediate values and conditions that may not be behaving as intended.
4. Quick and Temporary
Unlike more complex debugging tools like debuggers or logging frameworks, print statements are quick to implement and require no additional setup. For temporary issues or during early development stages, print debugging can save time.
5. Caveat: Clean Up Print Statements
While print statements are useful for debugging, remember to remove them once you’ve resolved the issue. Leaving print statements in production code can clutter the output and may expose sensitive information. To cultivate good coding habits and learn effective debugging strategies, consider enrolling in a Python Course in Bangalore, where you’ll receive guidance on best practices for writing clean, maintainable code.
6. Debugging Loops and Conditional Statements
Print statements are particularly helpful in debugging loops and conditionals. You can print loop counters or variable states within conditions to understand why certain code paths are not executed or why loops behave unexpectedly.
for i in range(5):
print(f”Loop iteration: {i}”)
if i == 3:
print(“Condition met!”)
7. Printing Exception Messages
By printing exception messages in your code, you can catch specific errors and understand what went wrong. This is especially useful when you’re not sure where an exception is occurring or why it is being raised.
try:
x = int(“string”)
except ValueError as e:
print(f”Caught an error: {e}”)
8. Tracking Function Calls
If your Python program consists of multiple functions, print statements can help track the sequence in which functions are called. This is useful for debugging complex workflows and ensuring that the function calls occur in the intended order.
def func1():
print(“Function 1 called”)
def func2():
print(“Function 2 called”)
func1()
func2()
9. Checking Data Structures
Print statements can also help you inspect data structures like lists, dictionaries, or sets. Printing these structures allows you to see how they change as your program executes, making it easier to debug issues related to data manipulation.
my_list = [1, 2, 3]
print(f”Initial list: {my_list}”)
my_list.append(4)
print(f”Updated list: {my_list}”)
10. Understanding Recursion Depth
For recursive functions, print statements are helpful in visualizing the depth of recursion. By printing the function’s inputs at each recursion level, you can better understand how the recursion unfolds.
def recursive_func(n):
print(f”Recursion depth: {n}”)
if n > 0:
recursive_func(n-1)
recursive_func(3)
11. Ensuring Code Coverage
Using print statements in sections of code that might not always execute—like within rare conditionals or edge cases—ensures that all parts of your code are covered. This practice helps catch hidden bugs or misbehaviors in rarely tested branches of your code.